Today, I messed around with making a webpage where you can play with balloons. Here’s how it went down.
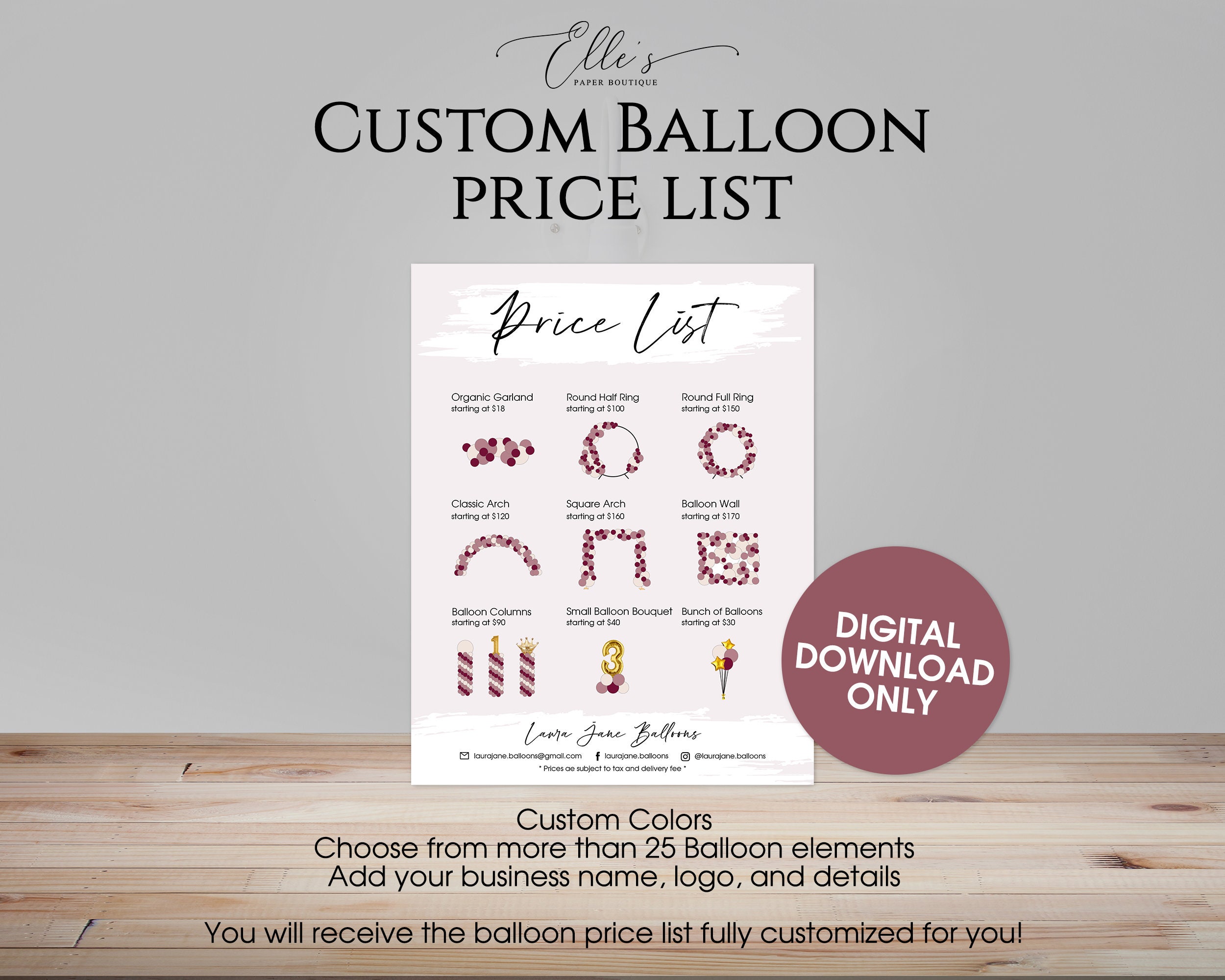
Getting Started
First, I opened up my code editor and made a new HTML file. I added the basic stuff like the <!DOCTYPE html>
, <html>
, <head>
, and <body>
tags. I also added a title tag for the webpage, named it “Balloons Fun”.
Adding Some Style
Next, I wanted to make the page look a bit nicer, so I added some CSS. I created a <style>
tag inside the <head>
section. I set the background color to a light blue and centered everything using text-align: center;
.
Creating the Balloons
Now for the fun part – the balloons! I added a few <div>
elements inside the <body>
and gave them a class called “balloon”. In the CSS, I styled these divs to look like balloons. I made them round using border-radius: 50%;
, gave them different colors, and set their position to absolute
so I could move them around freely.
Making Them Interactive
To make the balloons interactive, I added some JavaScript. I wrapped everything in a <script>
tag at the end of my <body>
. First, I got all the balloon elements using *('.balloon')
.
- I added an event listener for
mouseover
to each balloon. When you hover over a balloon, it changes color. - I also added an event listener for
click
. When you click a balloon, it “pops” – I just removed the element from the DOM using .
Adding More Balloons
It would be boring with just a few balloons, right? So, I wrote a loop in JavaScript to create a bunch more. I set up a loop that runs 50 times, and each time it creates a new <div>
, gives it the “balloon” class, assigns a random color, size, and position, and then appends it to the <body>
. I also added the same event listeners to these new balloons.
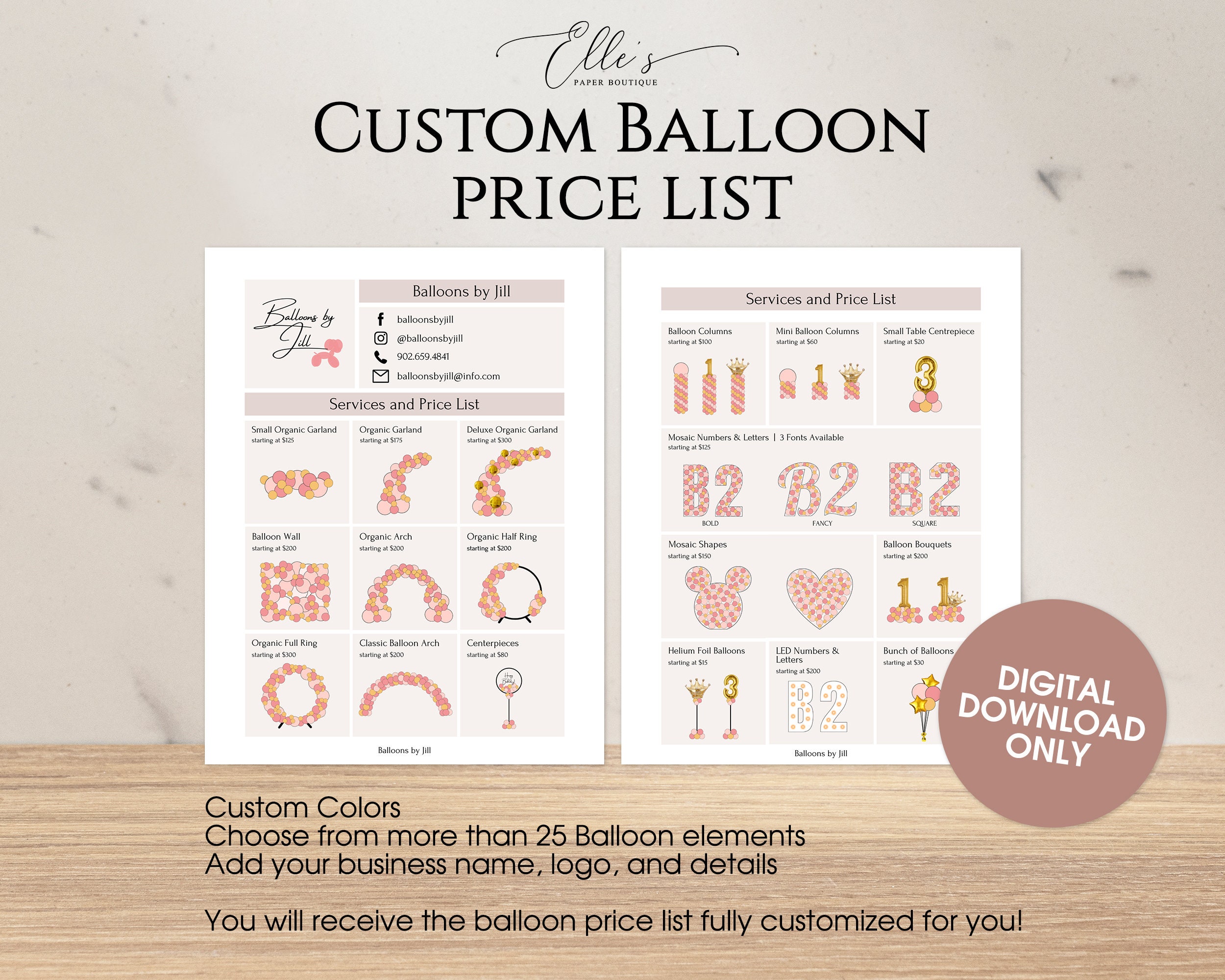
Final Touches
Finally, I added a little button at the top that says “Add More Balloons”. I gave this button an onclick
event that calls a function to add, say, 10 more balloons to the page. This way, you can keep adding balloons as long as you want!
And that’s it! I now have a simple webpage where you can play with a bunch of colorful, interactive balloons. It was a fun little project, and I learned a lot about handling DOM elements and events in JavaScript. Hope you enjoyed this little walkthrough!